Day 06 - Prepare to Step Into GUI¶
Today, we start to draw rectangles in C++.
Assembly Codes
C++ Code
Linking Template
Makefile
C++ Implementation¶
So far, the VGA is configured to be in Text mode. Draw a rectangle is equivalent to print colored empty charactor at specific locations on the screen. The core code reads
int main(){
/* some other codes */
boxfill8(p, SCREENX, RED, 0, 5, 10, 12); // from (0, 5) to (10, 12)
boxfill8(p, SCREENX, GREEN, 50, 12, 70, 23);
boxfill8(p, SCREENX, DARKBLUE, 79, 23, 80, 24);
return 0;
}
void boxfill8(char *vram, int xsize, unsigned char c, int x0, int y0, int x1, int y1){
/*
* This function will draw a box (rectangle) on the screen which has width xsize
* c is for color
* (x0, y0) and (x1, y1) are two diagonals of the box. These are absolute coordinates
*/
int x, y;
if(y0 > y1){
y = y0; y0 = y1; y1 = y;
}
if(x0 > x1){
x = x0; x0 = x1; x1 = x;
}
for (y = y0; y < y1; y++){
for(x = x0; x < x1; x++){
vram[y * xsize*2 + x*2+1] = c<<4;
}
}
}
Result¶
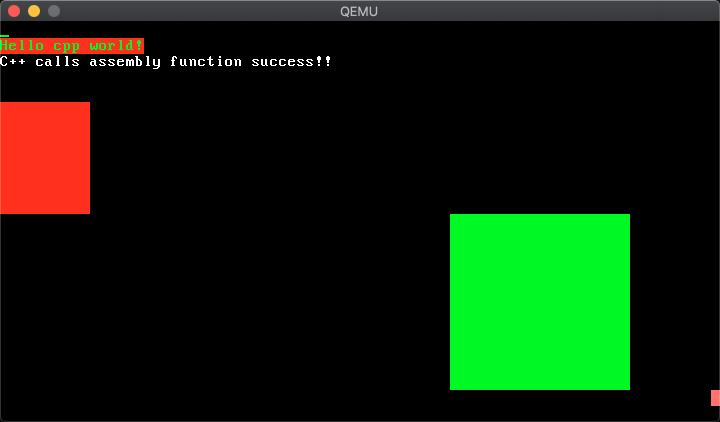