3. Docker Interactions¶
Before moving to a more sophisticate tutorials, it may be helpful to introduce trick/shortcuts/methods to interact with the docker container.
3.1. Attach and Detach¶
docker run -d ubuntu:15.10 /bin/bash -c "while true;do echo hello world;sleep 1; done"
The above command has a flag -d
which means detach the container output. Therefore, even though the container keeps printing out “hello world” once per second, we cannot see it in the terminal. The execution result is a container id.
3.1.1. Detach¶
The official document says that you can use ctrl-p and then ctrl-q to detach a running container. But it doesn’t work for my MAC OS. One simple solution for my is to close the terminal. The container will still run in the background.
3.1.2. Attach¶
# docker attach <container id or container name>
docker attach quirky_heisenberg
3.2. Connect Docker Container¶
During the development, you may need to modify some configuration or codes in the docker container. Then, you need to access the file system of the docker container.
3.2.1. Via VSCode (easy)¶
Install two plugins in VSCode named Docker and remote container.
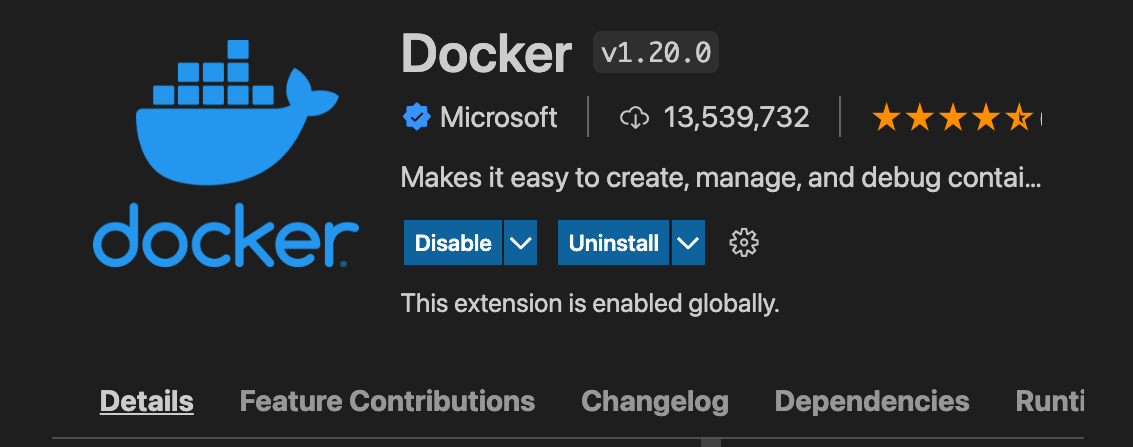
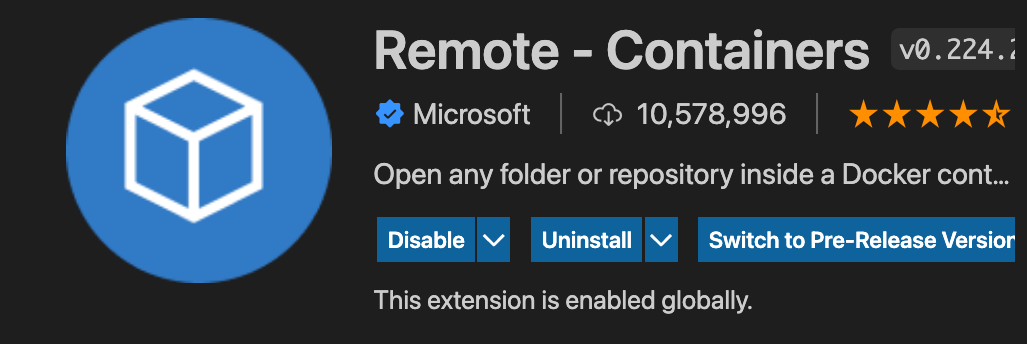
Then, press cmd+shift+p and select the item named Remote-Containers: Attach to Running Container. Then you can specify which running container you would like to access.


Then, the vscode can access the container’s file system, and you can modify the file you like.
3.2.2. In Terminal¶
Get the container id or name using docker container ls
.
Then execute the following code to attach the terminal
docker exec -it <container id or name> /bin/bash
3.3. Copy Files From and To A Docker Container¶
The command you want to use is docker cp
. The usage is pretty simple
# copy from container to host
docker cp <container id/name>:<path to file/directory> <local path>
# copy from host to container
docker cp <local path of file/directory> <container id/name>:<path>
# For example, copy test.py from the container to local machine
docker cp quirky_heisenberg:~/test.py ~/
# copy test2.py from local machine to the container
docker cp test2.py quirky_heisenberg:~/